Organizing files in front-end projects is essential for maintaining clear and efficient code. Good practices help developers find, modify, and manage files quickly, which speeds up development and reduces errors. Without a solid structure, projects can become confusing and hard to scale.
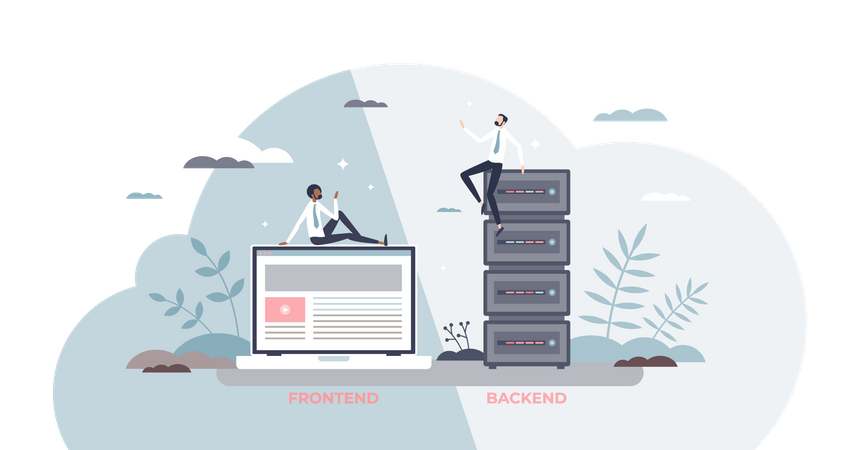
A well-organized project divides code into meaningful folders, groups related files together, and separates different types of resources. This approach makes it easier to work in teams and supports better testing and version control. Clear naming and consistent file placement play a big role in keeping the project clean and manageable.
Key Takeways
- A clear folder structure improves code clarity and development speed.
- Grouping related files and resources reduces confusion.
- Consistent naming and organization support project growth and teamwork.
Structuring the Root Directory
A well-organized root directory helps keep a front-end project clear and manageable. It simplifies finding files and reduces confusion as the project grows. Proper separation, naming, and management of configuration files are key to achieving this.
Separation of Concerns
Files in the root directory should be grouped by their purpose. For example, source code belongs in a folder like src
. Static assets such as images and fonts should go into an assets
or public
folder.
This separation keeps code clean and makes it easy to locate specific files. It also helps new developers understand the project faster. Avoid mixing different file types in one folder, as this can cause clutter.
Consistent Naming Conventions
Using consistent naming conventions for files and folders prevents errors and confusion. Names should be clear and descriptive, using lowercase letters with hyphens or camelCase. For example, use user-profile.js
or userProfile.js
.
Avoid spaces and special characters. Keep file extensions meaningful like .js
, .css
, or .json
. Consistency in naming allows tools and developers to work more smoothly across the project.
Managing Configuration Files
Configuration files should be easy to find and separated from the codebase. Place them in a dedicated folder like config
or keep them in the root if there are only a few.
Common files include .env
, package.json
, webpack.config.js
, and .gitignore
. It is important these files are named clearly and follow the standards of the tools they configure. Proper management avoids conflicts and makes setup easier for others.
Creating Feature-Based Folders
Feature-based folder structures group files by their purpose or functionality, not by file type. This approach helps developers find and update related code quickly. It also reduces confusion as projects grow in size.
Grouping by Feature
Files related to a single feature are kept together in one folder. This includes components, styles, tests, and helpers. For example, a “UserProfile” folder might hold UserProfile.js
, UserProfile.css
, and UserProfile.test.js
.
Grouping by feature makes the project easier to navigate. When a developer works on a feature, they don’t need to search many different folders. It also supports better collaboration, as teams can focus on specific feature folders.
Organizing Shared Components
Shared components used across features should be placed in a separate common or shared folder. These components are reusable parts like buttons, modals, or icons. Keeping them apart avoids duplication.
It is important to clearly name these shared folders, such as shared/
or common/
. This makes it easy to spot reusable code. Including documentation or README files here can explain how the shared items should be used.
Avoiding Deeply Nested Directories
Too many nested folders can make the project harder to understand and slow down navigation. Front-end projects should limit folder depth to about 2-3 levels.
Instead of creating multiple subfolders within each feature folder, combine related files in fewer folders. This keeps the structure flat and easier to scan. Tools like automatic imports or search can handle more complex file setups if needed.
Handling Assets and Static Files
Managing assets carefully can improve project clarity and loading speed. Grouping similar files together and keeping names clear helps avoid confusion and makes maintenance easier.
Organizing Images and Fonts
Images should be stored in a dedicated folder named something like /assets/images
or /static/images
. Fonts belong in a separate folder such as /assets/fonts
. This separation keeps file types clear and easy to find.
Use descriptive file names that reflect the image content or font style. For example, logo-primary.svg
or open-sans-bold.woff2
provide clear context.
Avoid mixing different file types in one folder. This helps the team find and update specific assets without hunting through unrelated files.
Optimizing Asset Folder Structure
Keep the asset folder structure shallow. Deeply nested folders make files harder to locate. A good structure looks like this:
Folder | Contents |
---|---|
/assets/images | PNG, JPG, SVG files |
/assets/fonts | WOFF, TTF font files |
Use subfolders only when necessary, such as grouping images by page or feature (/assets/images/home
). This keeps the structure tidy.
Static files should be organized by type and use case to speed up loading. Compress images and remove unused fonts to reduce project size and improve performance.
Managing Components Effectively
Organizing components clearly helps make a project easier to manage and scale. Each part should be easy to find, update, and reuse without confusion.
Reusable UI Components
Reusable components save time and reduce mistakes. They are built to work in different parts of the app without changing their code much.
Developers should place shared components in a common folder named something like components
or ui
. Each component should have its own folder with files for its code and tests. Naming folders and files clearly, for example, Button
or InputField
, helps other team members understand what they do quickly.
Using props and callbacks keeps components flexible. Writing small, focused components allows combining them to build more complex UI elements. Keeping lifecycle methods and side effects minimal makes reusing components safer.
Component-Level Styles
Styling should be close to the component it affects. This keeps styles scoped and prevents conflicts between components.
Using CSS Modules or styled-components is common. These methods attach styles only to the component, avoiding global changes in a project. Storing style files like Button.module.css
or defining styles inside Button.jsx
keeps each UI piece self-contained.
Organizing styles inside the component folder or alongside its code helps developers find and change them fast. Consistent naming in styles also prevents errors, for example, using .button-primary
instead of vague class names like .blue
.
Organizing Stylesheets
Stylesheets should be structured to improve maintainability and ease of use. Clear separation between reusable, component-level styles and broader, global rules helps keep the project organized.
Modular CSS and SCSS Files
Modular CSS or SCSS files contain styles tied to specific components or features. This approach limits style scope, preventing unintended side effects across the project.
Files are usually named after the component, like button.scss
or card.css
. Each module file holds all necessary styles for that component, including layout, colors, and typography.
Using partials in SCSS (files starting with _
) helps import these modular styles without generating separate CSS files. This keeps the final output clean and improves build efficiency.
Benefits include easier debugging, faster updates, and better collaboration since developers focus only on the relevant styles. It also supports modular JavaScript frameworks well.
Theme and Global Styles
Theme files hold definitions for colors, fonts, spacing, and other design tokens used throughout the project. These files ensure consistent styling across components.
Global styles contain resets, typography defaults, and base element styles like body
and a
. These should be minimal to avoid conflicts with modular styles.
Organizing themes and globals into separate folders or files, such as theme.scss
and global.scss
, allows clear distinction from component styles.
Variables or CSS custom properties for themes make it easier to update the design system. This approach supports scalability and faster changes when redesigning or adding new themes.
Separating Utilities and Helpers
Organizing code by keeping utilities and helpers separate helps create cleaner and more maintainable Front-End projects. This practice promotes reuse and makes it easier to find and update specific functions. It also reduces clutter in main components.
Custom Hooks and Functions
Custom hooks in React should be stored in their own folder, often named hooks
or useHooks
. These hooks contain logic like state management, data fetching, or side effects. Keeping hooks separate prevents components from becoming too large or complex.
Functions that are used in many parts of the app but don’t affect the UI directly also belong here. For example, a hook for window resizing or user authentication logic should be in this folder. Naming hooks with a use
prefix clearly distinguishes them.
This setup allows easy imports like:
import useWindowSize from '../hooks/useWindowSize';
and prevents duplication of code.
Common Utility Files
Utilities are simple, reusable functions often stored in a utils
or helpers
folder. These functions handle tasks like formatting dates, manipulating strings, or math calculations.
A typical utilities folder might include:
formatDate.js
calculateSum.js
stringHelpers.js
Each file should export related functions. This keeps the project clean and makes it easy to find specific helpers.
For example, importing a utility might look like this:
import { formatDate } from '../utils/formatDate';
Keeping utilities focused and small improves testability and reduces bugs.
File Naming Best Practices
Good file names make it easier to find and understand files later. Clear rules help keep names consistent and reduce errors. This section explains how to name files for clarity using style rules and how to avoid confusing names.
Lowercase and Kebab-Case Conventions
Using lowercase letters keeps names simple and avoids case sensitivity issues on different systems. Kebab-case uses hyphens (-) to separate words, making names easier to read.
Examples:
main-header.js
user-profile.css
Kebab-case improves readability compared to camelCase or snake_case for file names. It is widely accepted in front-end projects, especially for CSS and JavaScript files.
Lowercase kebab-case also works better for URLs and command-line tools. Avoid spaces and special characters to prevent problems in some environments.
Avoiding Ambiguous Names
File names should clearly describe their content or function. Avoid vague names like temp.js
or data.txt
that don’t explain what the file does.
Use specific words that relate to the file’s role. For example, name a file that handles user forms as user-form-handler.js
instead of just form.js
.
Avoid using numbers or versions unless necessary, as they can cause confusion. If needed, place version info at the end, like component-v2.js
.
Clear names save time by reducing guesswork for anyone working on the project.
Optimizing for Scalability
Good file organization helps teams work together smoothly and makes it easier to add features later. A clear structure supports collaboration and prepares the project to grow without causing confusion.
Supporting Team Collaboration
Using a consistent folder and file naming system helps every team member find code quickly. It is important to separate components, assets, and utilities into distinct folders. This reduces overlap and makes code reviews simpler.
Teams should adopt clear README files and comments to explain folder purposes. Sharing coding standards in a document encourages uniformity. Tools like linters and formatters can enforce these rules automatically.
Version control systems like Git must be used with clear branching strategies. This prevents conflicts and keeps everyone’s work organized. Regularly updating shared documentation ensures new members can join easily.
Preparing for Project Growth
Projects tend to expand with new features and more complex logic. A scalable file structure anticipates this by grouping similar files logically, such as by feature or function. This prevents folders from becoming too large.
Splitting code into smaller, reusable components allows developers to build on existing work without rewriting. Using index files to export modules lets import paths stay clean and consistent.
Keeping third-party libraries separate from custom code reduces risks during upgrades. It is also useful to separate styles and scripts by feature or page to avoid clutter as the project grows.
Documentation and README Structure
Good documentation is key to keeping a Front-End project easy to understand and use. It must be clear, organized, and updated regularly. Descriptions should explain what each file and folder does to guide new and current developers quickly.
Maintaining Up-to-Date Guides
Documentation should be reviewed whenever changes happen in the project. This includes updates to dependencies, new features, or file reorganizations. Outdated guides confuse users and slow down development.
A best practice is to link documentation updates to code changes in pull requests. This encourages developers to fix or add instructions while working on the code. Version control systems like Git help track documentation revisions alongside project changes.
Including step-by-step instructions, command examples, and screenshots aids clarity. It should also mention any specific setup commands or environment variables needed for the project to run correctly.
Clear File and Folder Descriptions
Each folder and major file needs a short description explaining its content and purpose. This can be done inside the README or via separate markdown files dedicated to project structure.
Descriptions should answer:
- Why is this folder here?
- What type of files will be found?
- How do these files interact with other parts of the project?
For example:
Folder | Description |
---|---|
/components | Reusable UI elements |
/styles | CSS and style sheets |
/utils | Helper functions and constants |
Clear labeling makes navigation faster and reduces mistakes like editing the wrong file.
Implementing Testing Structure
A clear testing structure helps keep a project organized and makes tests easier to find. Tests usually live in a separate folder named tests
, __tests__
, or inside the source folder next to the related files.
Developers often group tests by feature or component. For example, a component’s test file might be named Component.test.js
and placed next to Component.js
. This keeps related code and tests close.
Using consistent naming for test files is important. Common patterns include:
Component.test.js
Component.spec.js
Component.test.tsx
Choosing one helps tools recognize test files quickly.
The structure should support different types of tests, such as:
Test Type | Location | Purpose |
---|---|---|
Unit Tests | Next to components or src | Test small parts alone |
Integration | In tests/integration folder | Test interactions |
End-to-End | In tests/e2e folder | Test user flows |
Tools like Jest, Testing Library, or Cypress follow these patterns well.
Keeping the testing structure simple and mirrored from the main codebase helps with maintaining and running tests. This approach reduces confusion when projects grow larger.
Version Control Organization
Version control helps track changes and manage code in front-end projects. It is important to keep the repository clean and easy to understand.
They should organize branches clearly. Common branches include main or master for stable code, and develop for new features. Feature branches should have descriptive names like feature/login-form
or bugfix/navbar-error
.
Commit messages must be clear and short. Each message should explain what was done without extra detail. For example, “Fix button alignment on mobile screens” is better than a vague message.
Files that do not need tracking should be listed in .gitignore
. This usually includes build files, dependencies in node_modules
, and environment files.
Tags and releases can mark important points in the project, like version updates. Tags should use consistent naming like v1.0.0
to keep everything organized.
A simple table for branch types could be:
Branch Type | Purpose | Example Name |
---|---|---|
Main | Stable production-ready | main |
Develop | Work in progress | develop |
Feature | New features | feature/user-profile |
Bugfix | Small fixes | bugfix/header-bug |
Good version control makes teamwork smoother and errors easier to fix.
read suggestion: How to avoid common accessibility errors in HTML and CSS
Leave a Reply to How to Create More Responsive Interfaces with Modern CSS Techniques for Enhanced User Experience – Euro Tones Cancel reply